はじめに
今まで「XAML」「バインディング」などの知識ゼロのままC#のコードだけでGUIを構築してきました。いよいよ「バインディング」について勉強を始めようと思います。「XAML」「バインディング」はセットだと考えている人もいるかもしれませんが決してそうではありません。「XAML」を使わないで「バインディング」を使用する方法を学習したので紹介させて頂きます。非常に簡単なサンプル
GUI部分とそうでない部分でファイルを分けてコードを書いています。ファイルは分けていますがクラスを新たに作らず同じクラス内に記述しています。GUI部分のファイル
using System.Windows; using System.Windows.Controls; namespace binding { public partial class MainWindow : Window { private void WindowSetting() { StackPanel mainPanel = new() { Orientation = Orientation.Vertical }; Content = mainPanel; Label lastName = new(); Label firstName = new(); lastName.SetBinding(Label.ContentProperty, "苗字"); firstName.SetBinding(Label.ContentProperty, "名前"); mainPanel.Children.Add(lastName); mainPanel.Children.Add(firstName); } } }
GUI部分でないファイル
using System.Windows; namespace binding { public partial class MainWindow : Window { public MainWindow() { InitializeComponent(); WindowSetting(); DataContext = new { 苗字 = "Yamada", 名前 = "Taro" }; } } }
結果
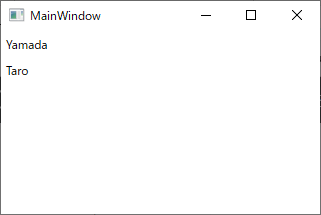
画像を表示させるサンプル
GUI部分のファイル
using System.Windows; using System.Windows.Controls; namespace imageShow { public partial class MainWindow : Window { private void WindowSetting() { Image imagePanel = new(); Content = imagePanel; imagePanel.SetBinding(Image.SourceProperty, "originalImage"); } } }
GUI部分でないファイル
using System; using System.Windows; using System.Windows.Media.Imaging; namespace imageShow { public partial class MainWindow : Window { public MainWindow() { InitializeComponent(); WindowSetting(); BitmapImage star = new(new Uri(AppDomain.CurrentDomain.BaseDirectory + "/star.png")); DataContext = new { originalImage = star }; } } }
結果
上の単純なコードで画像の縦横比は乱れませんでした。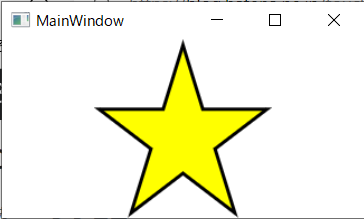
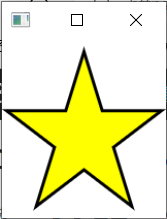
Windowsに含まれる「切り取り&スケッチ」で切り取った画像、きれいではありません(笑)。